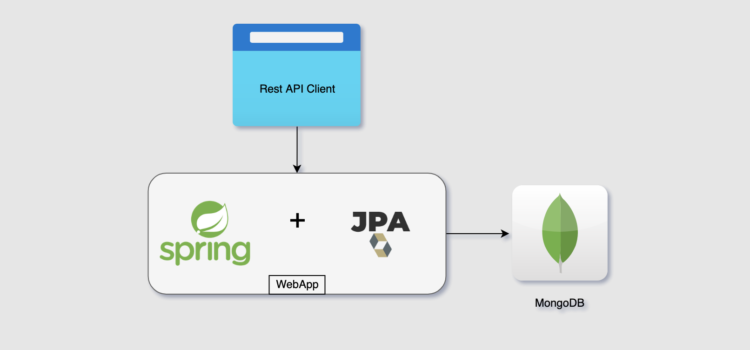
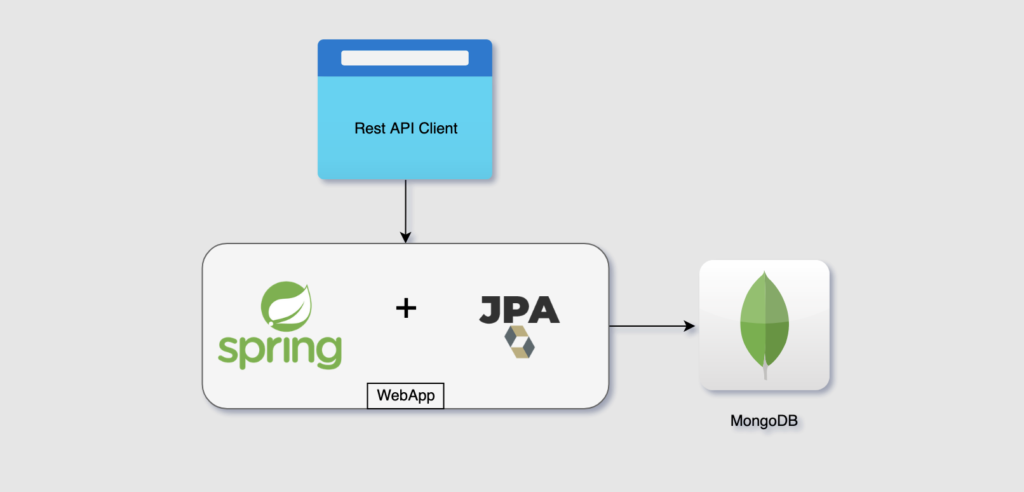
Table of Contents
1. Context
In this article, we have seen steps of creating a SpringBoot based imaginary TV program guide app that connects to PostgreSQL using JPA. The same code works for any relational database available in the market. We have to use the appropriate database driver and change the database configuration in the application.properties file. Using MongoDB
in place of PostgreSQL
is mostly the same. Here are the key differences:
- The ORM class is referred here as a document class and the annotation
@Document
is used instead of@Entity
. - The
@Id
field in the document and entity classes are handled differently - The Repository interface extends from
MongoRepositoty
instead ofJpaReposity
2. JPA Repository and Document
Here is the ProgramDocument representing the program
collection in MongoDB
:
package org.openapex.tvguide.model; import com.fasterxml.jackson.annotation.JsonIgnore; import lombok.AllArgsConstructor; import lombok.Getter; import lombok.NoArgsConstructor; import lombok.Setter; import org.springframework.data.annotation.Id; import org.springframework.data.mongodb.core.mapping.Document; import java.util.List; @Getter @Setter @NoArgsConstructor @AllArgsConstructor @Document(collection = "program") public class ProgramDocument { @Id @JsonIgnore private String id; private String title; private String type; private String genre; private String certification; private List<String> actors; private String director; private String producer; }
The Repository interface that extends from MongoRepository
and provides Java APIs for all sorts of database operations.
package org.openapex.tvguide.dbaccess.repository; import org.openapex.tvguide.model.ProgramDocument; import org.springframework.data.mongodb.repository.MongoRepository; import org.springframework.stereotype.Repository; @Repository public interface ProgramRepository extends MongoRepository<ProgramDocument, String> { }
The Controller and service classes are the same whether PostgreSQL is used or a MongoDB used for persistence.
3. Database Configuration
Database configuration in application.properties
file:
spring.data.mongodb.host=localhost spring.data.mongodb.port=27017 spring.data.mongodb.database=tvguide
The database script to create a collection named program
and add some sample programs
:
use tvguide; db.createCollection('program'); db.program.insertMany([ { 'title':'Casablanca', 'genre':'Movie', }, { 'title':'Friends', 'genre':'Comedy', } ]);
4. Conclusion
The Controller and service classes are the same whether PostgreSQL
is used or MongoDB
is used for persistence. In addition to channels endpoint, a new REST endpoint is exposed based on program data in MongoDB
:
- GET
/guide/programs
– lists all programs
The full code of this program guide app is available at GitHub: tvguide
Join our list to get instant access to new articles and weekly newsletter.