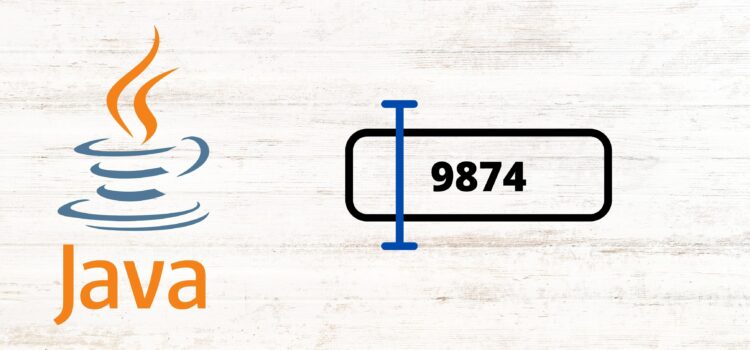
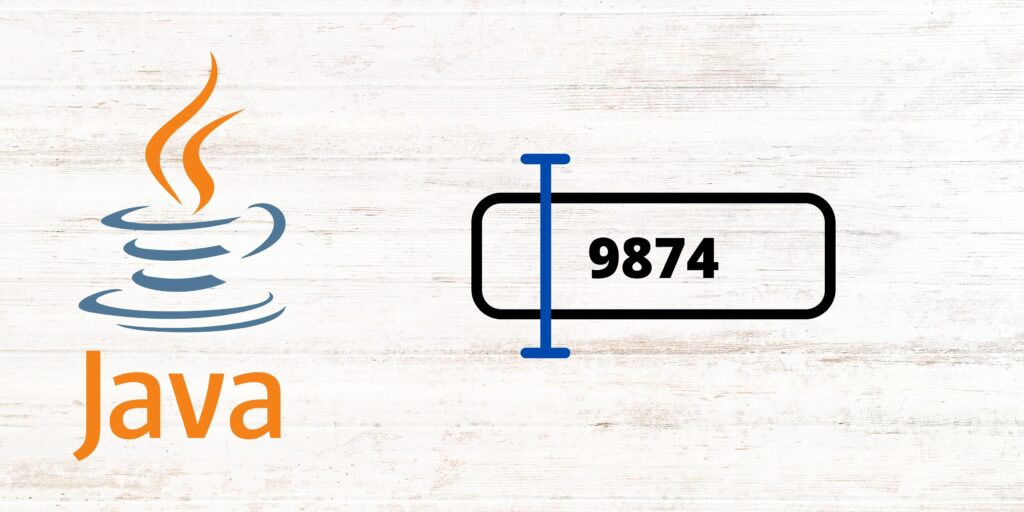
The JTextField
component in javax.swing
package is a general-purpose text field that accepts any input character. Often applications need to restrict the text field to accept only a certain type of inputs. One such use case is – the text field only allows numbers without decimal points (integers) and also it must restrict the length of characters.
We are going to use javax.swing.text.DocumenntFilter
for this purpose. The document filter attached to the text field would perform validations while inserting ore replacing text in the text field.
Table of Contents
1. Filter to Allow Only Numeric Input of Limited Length
NumericAndLengthFilter
takes length argument in the constructor to impose input length restriction on JTextField
. Both insertString()
and replace()
methods are overridden so that not only key input, but pasted data from the clipboard also can be validated. Both the methods calculate the effective length considering the current data on the field and the new incoming data. If the length is within the permissible range, the input data is then validated for the numeric check using a simple number test method.
/** * A document filter for numeric and length check. */ private class NumericAndLengthFilter extends DocumentFilter { /** * Number of characters allowed. */ private int length = 0; /** * Restricts the number of charcacters can be entered by given length. * * @param length Number of characters allowed. */ public NumericAndLengthFilter(int length) { this.length = length; } @Override public void insertString(FilterBypass fb, int offset, String string, AttributeSet attr) throws BadLocationException { if (isNumeric(string)) { if (this.length > 0 && fb.getDocument().getLength() + string.length() > this.length) { return; } super.insertString(fb, offset, string, attr); } } @Override public void replace(FilterBypass fb, int offset, int length, String text, AttributeSet attrs) throws BadLocationException { if (isNumeric(text)) { if (this.length > 0 && fb.getDocument().getLength() + text.length() > this.length) { return; } super.insertString(fb, offset, text, attrs); } } }
The below method checks whether the supplied string is a number. This method does not even allow decimal point. However, the implementation of these methods can be enhanced further according to the requirement.
/** * This method tests whether given text can be represented as number. * This method can be enhanced further for specific needs. * * @param text Input text. * @return {@code true} if given string can be converted to number; otherwise * returns {@code false}. */ private boolean isNumeric(String text) { if (text == null || text.trim().equals("")) { return false; } for (int iCount = 0; iCount < text.length(); iCount++) { if (!Character.isDigit(text.charAt(iCount))) { return false; } } return true; }
In case typed in or copied data is invalid, insert or replace operation is discarded. So, now we have successfully implemented a numeric text field with the help of a javax.swing.text.DocumentFilter
.
2. Runner Code
In createUI()
method, as usual, an instance of JTextField
is created and then added to a JPanel
. To put restrictions on the JTextField
, we use javax.swing.text.DocumentFilter
. DocumentFilter
provides a mechanism to apply a filter on document associated with swing text components such as JTextField
.
/** * NumericTextField allows only numbers in the text field and not even decimal * points. Also, it restricts the length of input. */ public class NumericTextField { /** * Creates the UI with the text field that accepts numeric data of specified * length. */ public void createUI() { final JFrame frame = new JFrame("NumericTextField"); frame.setSize(400, 400); JPanel panel = new JPanel(); JLabel label = new JLabel("Enter some data (only numbers would be allowed):"); final JTextField textField = new JTextField(30); // Add the document filter to text field for numeric and length check. ((AbstractDocument) textField.getDocument()).setDocumentFilter(new NumericAndLengthFilter( 5)); panel.add(label); panel.add(textField); frame.getContentPane().add(panel); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } /** * Entry point of the application. * * @param args Input arguments. */ public static void main(String[] args) { final NumericTextField test = new NumericTextField(); SwingUtilities.invokeLater(new Runnable() { public void run() { test.createUI(); } }); } }
3. Download
You may download the complete source code from here: NumericTextField.java
Join our list to get instant access to new articles and weekly newsletter.