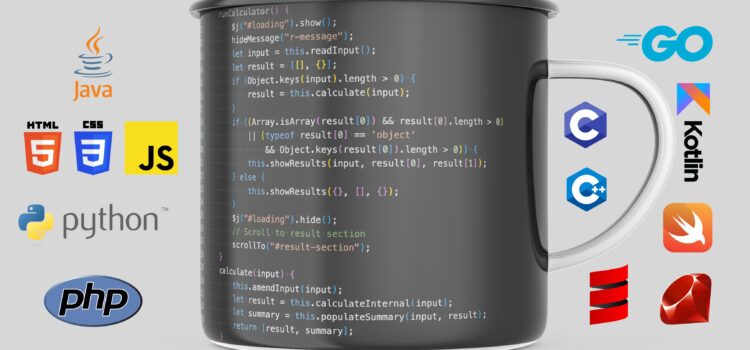
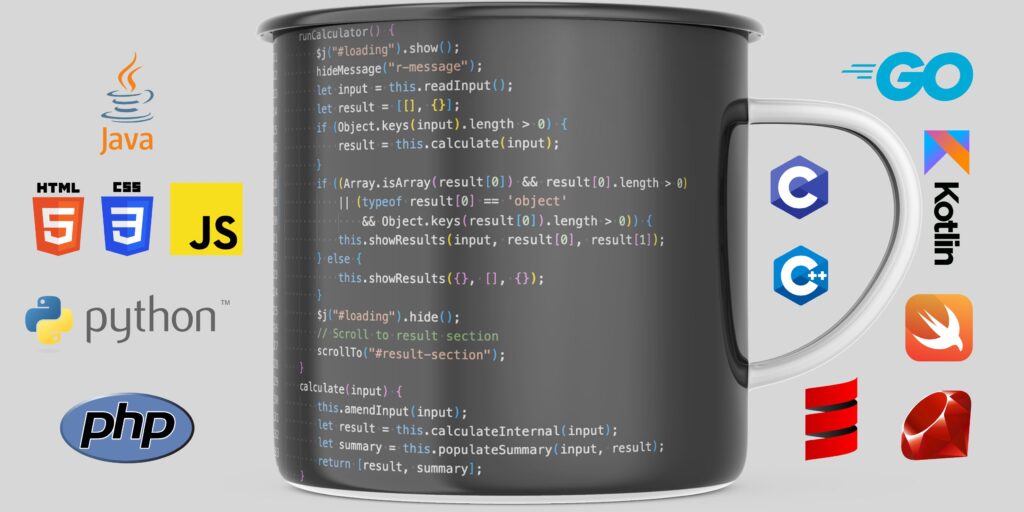
To most programmers, procedure, function, and method are the same thing. It is a callable, reusable code block that optionally takes some input, performs one or more operations, and optionally returns some output. However, there are subtle differences and definitions vary from language to language. We’ll try to keep the comparison as generic as possible.
Procedure Vs. Function Vs. Method
Procedure
- Reusable and callable code block
- Does not return value
- Intended to cause side effect
- Dedicated keyword procedure to create a procedure in Pascal and stored procedure in SQL
Function
- Reusable and callable code block
- Returns value, but that’s optional (void)
- May or may not cause side effects
- Programming languages have different keywords to create a function: function, def, fun, etc.
Method
- Reusable and callable code block
- Returns value, but that’s optional (void)
- May or may not create side effects
- Same keywords as used in function creation
- Associated with an object.
- In Object-oriented languages, member functions of a class are called methods.
A procedure is a function that does not return a value. In older programming languages such as Pascal, a procedure is defined with a dedicated keyword procedure
. Procedures are meant to perform operations with supplied inputs and cause side effects (e.g. logging).
A function is a general term used for a reusable code block. In the old days, functions were meant to perform calculations with supplied inputs and return the calculated results without causing side effects. However, in modern-day programming languages, we don’t distinguish between functions and procedures. A function may or may not return a value (void
) and may cause side effects.
A method is a function that is associated with and acts on objects in object-oriented programming languages. Methods are defined within a class and may or may not return a value (void
).
Let us create a reusable code block that takes principal, interest rate, and time as input parameters to calculate simple interest and return it. Here is a JavaScript function
to compute simple interest:
function computeInterest(principal, rate, time){ return principal * rate * time; }
Here is a Python function
to compute simple interest:
def computeInterest(principal, rate, time): return principal * rate * time;
Here is a Java method
to compute simple interest:
public double computeInterest(double principal, double rate, double time){ return principal * rate * time; }
Join our list to get instant access to new articles and weekly newsletter.